public handleSelectTaskItem(event, taskItem:any) {
designersdairy
Search This Blog
Tuesday, June 13, 2023
Validating to select in sequencial order using angular
Friday, April 28, 2023
Angular- validating the form field using adding 4 digits number
To prevent the letter "e" from being entered as a valid character in an input field of type "number", you can use the "onkeydown" event and check if the "e" key is pressed. If the "e" key is pressed, you can prevent the default behavior of the input field, which is to allow the "e" key to be entered as part of a scientific notation number.
Here's an example of how to prevent the letter "e" from being entered in an input field of type "number" using the "onkeydown" event:
<input type="number" formControlName="frequency" class="form-control" [readOnly]="!isEditable" inputmode="numeric" pattern="[0-9]*" onKeyPress="if(this.value.length === 4 || event.key === 'e') return false;" />
Wednesday, November 30, 2022
How to create a nested array
Step1:
To create nested array
Step2:
Excepting the Output of Nested Array
{
requestDate : "16-03-2023",
copyshPdRec : 1,
copyshcomment : 2
requestDate : "16-03-2023",
copyshPdRec : 1,
copyshcomment : 2
},
requestDate : "16-03-2023",
copyshPdRec : 1,
copyshcomment : 2
}]
}
Wednesday, September 21, 2022
Array iterators and working through nested objects and arrays - https://medium.com/@vshan0816/array-iterators-and-working-through-nested-objects-and-arrays-81e3f762e2ad
Array iterators and working through nested objects and arrays
One of the more challenging concepts that we’ve learned while studying Javascript is iteration. Iteration is basically going through an object or array and performing a function on all the individual elements of the the object or array to change the data structures in a certain way. Objects are organized by key-value pairs, while array’s are organized by indexes. The index of the first element in an array starts at zero or [0], and the next is [1] and so on.
The most basic way to iterate over objects and arrays are to use loops. For objects, we use what is called a for…in loop.
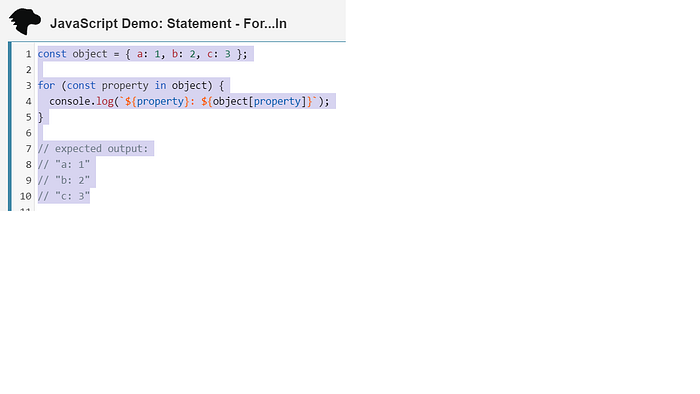
MDN gives us the following example. Notice that the for…in loop is iterating through the individual keys of the object. Here each key is assigned the variable, “property”. The for…in loop is trying to console.log both the key and the corresponding value so there are some additional steps. In order to access the values of each key, we have to use bracket notation, as seen by “object[property]”. We used bracket notation instead of dot notation because “property” is a variable and not the name of a key. Also notice the usage of the backticks and the interpolation. The backticks allow us add additional string elements such as the colon, and the interpolation inserts the variables into the string. Once again, we are iterating over each key within the object in order.
The most basic way to iterate over an array is using a for…of loop.
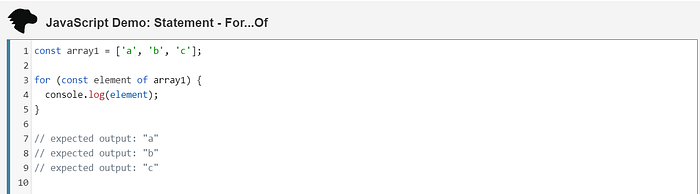
MDN gives the following example. A for…of loop allows you to iterate through an array or array-like object including a string in the order of each index. Here the for…of loop is logging each element of array1 into the console as can be seen by lines 7–9.
The forEach() method can be used similarly to a for of loop but it’s only used on arrays. The syntax is also different

MDN provides the follow example. The method forEach() is invoked on the array, and the parameter is a callback function that targets each individual element of the array in the order of their index.
Other array methods include find, filter, map, and reduce. Find returns the first element that matches the condition given in the callback. If no elements match the condition, undefined is returned. Filter returns a new array with all the elements that match the condition given in the call back. Map creates a new array with all the elements of the original array after they have been changed by a function given in the callback. Reduce, aggregates usually an array of numbers into a total.
Flatiron has a lab called hashketball that gets students to practice these concepts using a nested object with arrays. The nested object given is as follows.

gameObject is a function that contains the nested objects with the keys of home and away. Home and away contain an object made up of keys of teamName colors and players. teamName contains a string. Colors contains an array of colors. Players contains an array of objects with keys of playerName, number, shoe, points, rebounds, assists, steals, blocks, and slamDunks.
One of the challenges asks to build a function called numPointsScored that takes in an argument of a player’s name and returns the number of points scored for that player.
So in order to this we must first realize that we need to use the find function because it will match the player name in the argument, with the player name in the array.
We also need an array with all the playerNames that the find function will be invoked on. In the function the playerNames are divided into Away and Home, so the most efficient solution would require us to combine them into a single array.
One of my initial thoughts on how to solve the playerName solution was to create a new empty array and push all the playerNames into the array using an iterator. However we still need the information that is tied to the players key because we need to return the player points.
Instead of pushing the players into a new array we can do the same thing using a spread operator. The spread operator or “…” will allow us to copy an array and add it to a new array.
const players = () => [...gameObject().home.players, ...gameObject().away.players]
We set a variable to the new array called players. The dot notation allows us to access the property players. Players is within home and away which is within gameObject(). This combines the players from home and away which contains an array of objects with the information we need of playerName and points.
In order to match the playerNames with the argument, we need to use the find method as follows.
const player = (name) => players().find(player => player.playerName === name)
The return value is the object under players which contains the playerName, number, shoe, points, rebounds, assists, steals, blocks, and slamDunks.
In order to return the points of the matching player, we use the following code.
const numPointsScored = (name) => {
return player(name).points
}
This runs the find function that we defined as “player” on the “name” which is the argument, and returns the points of the matching playerName.
There are even tougher challenges in the lab such as
Which player has the most points? Call the function mostPointsScored.
Which team has the most points? Call the function winningTeam.
Which player has the longest name? Call the function playerWithLongestName.
These can be tackled with a similar approach. Ask yourself what iterator methods you would need, how would you organize the information, how you will access the information you need within the nested object, and how you would make the coding concise. I will not be going over how to solve the whole lab to not spoil it. As you can see, iteration can be a tough topic especially when it involves nested information.
Tricks to Loop Through an Array of Objects in JavaScript - https://www.webmound.com/javascript-loop-array-of-objects/
We loop through an array of objects in JavaScript for many reasons. For example, you can iterate and find an object from an array of objects using JavaScript based on some condition.
The forEach()
method can be used to loop through the array of objects in JavaScript. It gives access to each object present inside an array. There are other statements like for
and for…of
in JavaScript that can do the same thing.
You don't need any special methods or techniques to iterate over an array of objects. You can use the regular JavaScript array methods.
const people = [
{
name: 'john',
age: 35,
},
{
name: 'david',
age: 27,
},
{
name: 'kavin',
age: 35,
},
{
name: 'william',
age: 43,
},
{
name: 'jimmie',
age: 21,
},
];
I will use this "people" array to give you examples though out this article.
You will see 3 ways to loop over this array of objects. You will also learn when you should choose which one at the end of the post.

Use forEach() to Loop Over an Array of Objects
The forEach()
method is one of the easiest ways to iterate over an array of objects. This method accepts a callback function and executes that function for each item in the array.
people.forEach((person) => {
for (const key in person) {
console.log(`${key}: ${person[key]}`);
}
});
Output:
name: john
age: 35
name: david
age: 27
name: kavin
age: 35
name: william
age: 43
name: jimmie
age: 21
You also have access to each object and its index as the function parameters. I am looping over the people
array and getting every object in the person
parameter.
Now, you can use this object however you want. I am using the for...in
statement to loop through the object properties in JavaScript.
Use for Loop to Iterate Over an Array of Objects
JavaScript has a traditional the for
statement that can create a loop and give you access to all items inside an array. Therefore, you can use this statement to loop over an array of objects.
for (let i = 0; i < people.length; i++) {
console.log(people[i].name);
}
Output:
john
david
kavin
william
jimmie
It is very easy to the for
statement in JavaScript. You start from index 0 and continue the loop until the index is less than the length of the people
array.
Inside the loop, you have the current index value that can give you the current object. I am printing the name of each person from the array.
But you can perform any operation you want. Like you can shuffle a JavaScript array by using this technique.
Use for…of to Loop Through an Array of Objects
The for...of
statement is used to execute a loop over a JavaScript array. It is similar to the traditional for
statement but has an easy syntax.
It will loop through an array of objects and give you access to the objects directly instead of the index.
for (const person of people) {
console.log(`${person.name} is ${person.age} years old.`);
}
Output:
john is 35 years old.
david is 27 years old.
kavin is 35 years old.
william is 43 years old.
jimmie is 21 years old.
I am creating the loop over the people
array and getting each object in the person
variable. Now, you can check the objects and remove items from the array very easily.
Conclusion
You have seen 3 ways to iterate over an array of objects. The forEach()
method is very easy to use because of its simple syntax. But if you consider the performance then it may not be the best choice.
Note: The traditional
for
statement is much faster than theforEach()
method in JavaScript. If your application needs performace optimization consider usingfor
loop instead of theforEach()
method.
Another advantage of using for
and for...of
is that you can apply the break and continue statements of JavaScript for some conditions.
But as I have seen so far, it really doesn't matter 99% of the time. People use the forEach()
method in most of their applications. So if you are also using this method, it's not the end of the world.
So, you can apply any of these techniques to loop through an array of objects in JavaScript for your application.
Validating to select in sequencial order using angular
< input type = "checkbox" (change) = "handleSelectTaskItem($event, taskItem)" [checked] = " taskItem . i...
-
Welcome to designers blog here i have posted how to set xamp open localhost:8080 instead of just oepning localhost and Making the change ...
-
< input type = "checkbox" (change) = "handleSelectTaskItem($event, taskItem)" [checked] = " taskItem . i...
-
Step1: Welcome to designers bog Step1: SqlConnection con = new SqlConnection(@"data source=VAIO\SQLEXPRESS; database=Sam...